- Published on
Atari FontMaker
Atari FontMaker and its conversion from Delphi to C#
The Atari FontMaker is one of those tools that you find when looking for something modern to do your font/character set design. There are plenty of font editors for the Atari, but they all run on the 8-bit hardware, yeah sure you can run them in Altirra but there is something to be said about mouse control, big screens and lots of screen real estate.
I've been using the tool since 2021 when I started writing Retro Dschump, even asking MatoSimi to add a feature to detect duplicate characters in a font. This was added quickly and efficiently and the tool became even better.
Starting on a new project I needed to design new fonts and just did not have enough characters with the two supported fonts. Since the code is open source I started looking into adding the features I needed. That route quickly turned into a flat NO. Written in Delphi I just did not want to go down the route of learning yet another language. On closer inspection of the source code a little folder caught my attention:
/csharp 2021-05-27 matosimi [r67] added converted code to C# into csharp director...
This turned out to be the auto converted Delphi code. The result was not runnable but it have a basic C# code base.
Reading through the files it did not look difficult to get the converted code to work. Some graphics drawing functions had to be written but it was quick and easy to get the basic functionality working. But as it quickly turned out the auto-converted code was a mess.
The decision was taken to start from scratch. I liked the interface and the functionality and wanted to keep the soul of the Atari FontMaker the same. So I fired up Visual Studio 2022, clicked the 'Create new project' button, clicked the 'Windows Forms App' button and created the base of the new version of Atari FontMaker.
Step 1: GUI
The first step was to get the current GUI definition copied over to the new project. This was not too difficult. Visual Studio's
gui editor is ok if not exactly fast. The biggest issue was setting up where the event handlers will be located in the source code.
I like to break up my code into various topic specific source files, but the GUI editor does not like event handlers sitting in various
source files. In the end all event handler functions were moved to FontMakerForm.cs
.
Step 2: Drawing routines
Second up was how to draw into the various PictureBox
elements in the GUI. Plenty of examples on how to do it:
using (var gr = Graphics.FromImage(img))
{
gr.FillRectangle(redBrush, new Rectangle(0, 0, img.Width, img.Height));
}
Easy but not very efficient. Don't take that the wrong way, plenty fast enough for the slowest PC just not good enough for a performance junkie.
The font data is defined as eight bytes per character where each bit is a pixel. The Delphi code did some heavy decoding where a byte was decoded into an array or pixels and then each pixel was drawn as a 2x2 rectangle.
All that code was ripped out and replaced with a method to directly draw to the memory container of a 24-bit bitmap.
var bmpData = BitmapFontBanks.LockBits(new Rectangle(0, 0, 512, 1024), ImageLockMode.WriteOnly, BitmapFontBanks.PixelFormat);
unsafe
{
...
}
BitmapFontBanks.UnlockBits(bmpData);
The 2x zoom and the mono and color draw operations are semi-unrolled loops and the individual bit or colors are accessed via bit operations.
Not sure if anybody will feel the difference but it makes for some fun coding.
Step 3: Extending the interface
With the basic functionality moved to C#, I informed MatoSimi about my porting efforts and he gave me write access to the project SourceForge repo. Have to say, I'm not a fan of SVN.
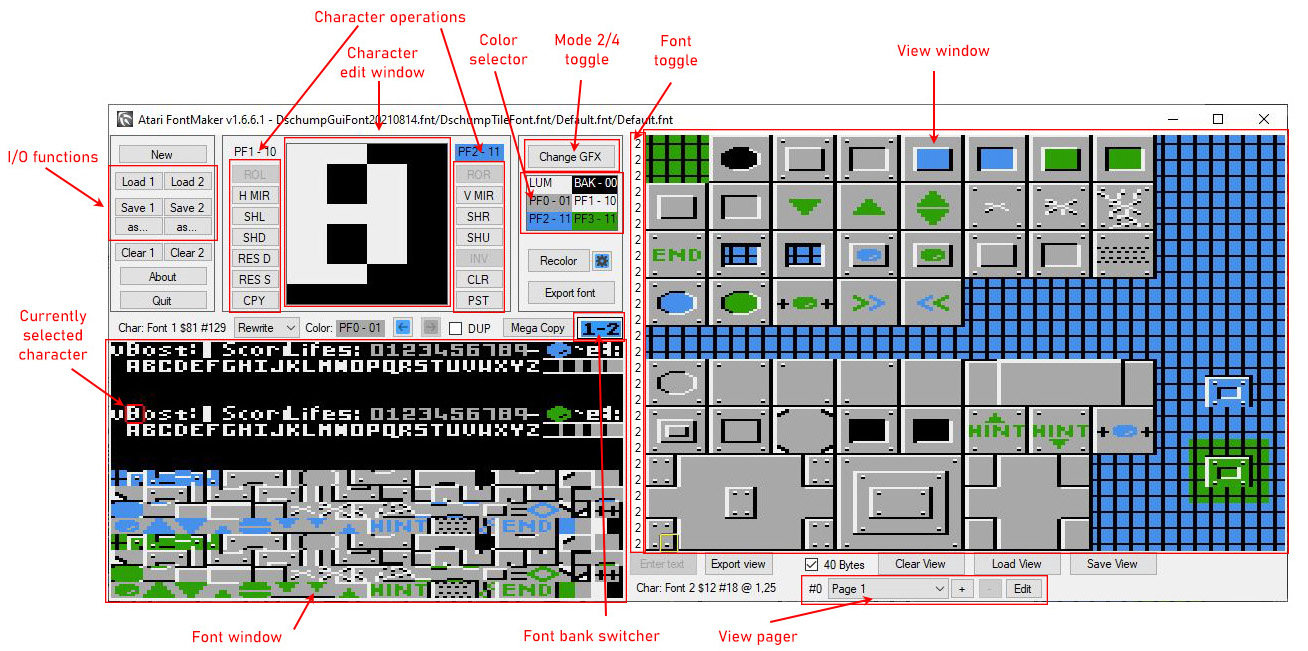
More fonts
First on my extension list was having more fonts. The original source code only handled 2 fonts in a Top-Bottom configuration. Not wanting to
break a good formula I kept the mechanism and just added a second bank of fonts. Atari FontMaker now supports 4 fonts. After making some space
next to the MegaCopy
button the font-bank button found its home.
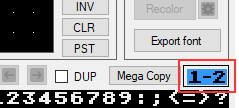
Any part of the GUI that allowed for font nr selection now handles all 4 fonts.
More view window area
The View Window is the perfect place to test your character/font creations. In RetroDschump I used the area to design my game tiles and very
quickly just ran out of space. There is only so much you can do with a 40x26 screen area. Again the gui was working and I did not want to
break the formula so I added pages to the view area. At the bottom of the screen, just below the view window, you can now find the page interface.
Use the +
button to add a duplicate copy of the current view. The pages will simply be numbered 1 though x. Hitting the Edit
button open a small
editor window that allows you to rename each page and change its sequence.
Now drawing a character animation and quickly flipping though the pages by using the mouse wheel over the page selector makes for a simple animation tester.
View exporter
Until now I had to use something like MapMaker to use my fonts to create data for inclusion in my projects. Some of the MapMaker functionality has been moved into the Atari FontMaker. You still only have a 40x26 screen, but now you can select any part of it and generate source code or export binary data.
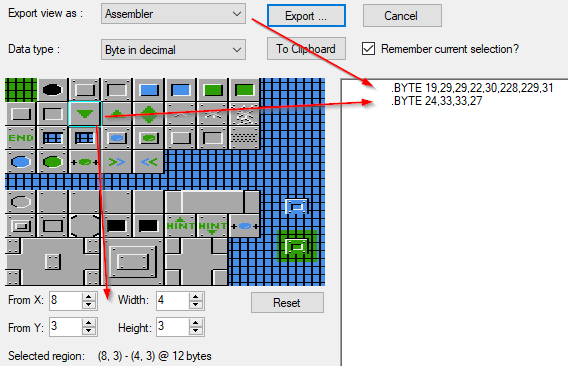
There is a picture of the current View Window which you can use to select all or parts for export. Data formats include Binary Data, Assembler, Action!, Basic, etc.
Future changes
Lets see what wishes the community has for this project. At least now the code should be more flexible and more people can help with new features. C# is not for everybody but I think there are more C# developers than Delphi developers out there.
Where can I find a copy?
The source code is now hosted on GitHub and can be found here: